Getting started with Nylas Scheduler
This feature is in public beta. It is generally stable, but some features might be added or changed before it is generally available.
In this tutorial, you'll learn how to install the Scheduler UI Components and set up Nylas Scheduler.
Before you begin
Before you start using the Scheduler UI Components, complete the following tasks:
- Sign up for a Nylas account.
- Sign in to the v3 Dashboard and create a Nylas application.
- Generate an API key for your application and save it.
- Authenticate an end user grant.
Install Scheduler UI Components
First, download and install the Scheduler UI Components in your project. The package includes both the Scheduling Component and the Scheduler Editor Component, and you can download it as either a set of web components (HTML/Vanilla JS) or React components.
npm install @nylas/web-elements@latest
npm install @nylas/react@latest
If you prefer, you can use unpkg
to directly include the web components in your HTML/Vanilla JS file.
<script type="module" src="https://unpkg.com/@nylas/web-elements@latest"></script>
Use Scheduler UI Components in your project
After you have them installed, you can either include the Scheduler UI web components in your HTML/Vanilla JS file, or import the React components to your React application.
The following examples show how you can include the Scheduling Component in your project.
<script
type="module"
src="./node_modules/@nylas/web-elements/dist/nylas-web-elements/nylas-web-elements.esm.js"
></script>
<html>
<body>
<nylas-scheduling></nylas-scheduling>
</body>
</html>
import React from 'react';
import { NylasScheduling } from '@nylas/react';
function App() {
return <NylasScheduling />;
}
export default App;
For more information, see the Scheduler UI Components reference documentation.
Create a configuration
Next, create a configuration for your first event. A configuration defines your preferred event settings and preferences. Nylas Scheduler stores Configuration objects in the Scheduler database, and loads them as Scheduling Pages in the Scheduler UI.
You can create a configuration in two ways:
Create a configuration using the Scheduler Editor
When you create a Scheduling Page using the Scheduler Editor in the UI, Nylas creates a corresponding configuration.
⚠️ You must have a working front-end UI to use the Scheduler Editor to create a configuration. Follow the Scheduler Quickstart guide to get a local development environment up and running.
To create a configuration using the Scheduler Editor, follow these steps:
- Set up the Scheduler Editor component.
- Set
nylasSessionsConfig
. The Scheduler Editor Component uses the Hosted authentication details innylasSessionsConfig
to interact with the Nylas APIs. - By default, Nylas creates a private configuration that requires a session. To create a public configuration without a session, set
requires_session_auth
tofalse
. See Scheduler configurations for more information.
- Set
The following example creates a public configuration.
<html>
<head>
<script type="module" src="https://unpkg.com/@nylas/web-elements@latest"></script>
</head>
<body>
<nylas-scheduler-editor />
<!-- Configure the Nylas Scheduler Editor Component -->
<script type="module">
const schedulerEditor = document.querySelector('nylas-scheduler-editor');
schedulerEditor.nylasSessionsConfig = {
clientId: 'NYLAS_CLIENT_ID', // Replace with your Nylas client ID
redirectUri: `${window.location.origin}/scheduler-editor`,
domain: 'https://api.us.nylas.com/v3', // or 'https://api.eu.nylas.com/v3' for EU data center
hosted: true,
accessType: 'offline',
}
schedulerEditor.defaultSchedulerConfigState = {
selectedConfiguration: {
requires_session_auth: false // Set to 'false' to create a public configuration
}
}
</script>
</body>
</html>
import React from 'react';
import { NylasSchedulerEditor } from "@nylas/react";
function App() {
return (
<NylasSchedulerEditor
nylasSessionsConfig={{
clientId: "<NYLAS_CLIENT_ID>", // Replace with your Nylas client ID
redirectUri: `${window.location.origin}/scheduler-editor`,
domain: "https://api.us.nylas.com/v3", // or 'https://api.eu.nylas.com/v3' for EU data center
hosted: true,
accessType: 'offline',
}}
defaultSchedulerConfigStoreState={{
selectedConfiguration: {
requires_session_auth: false // Set to 'false' to create a public configuration
}
}}
/>
);
}
export default App;
- Set up the Scheduling Component.
<html>
<head>
<script type="module" src="https://unpkg.com/@nylas/web-elements@latest"></script>
</head>
<body>
<nylas-scheduling />
<script type="module">
const nylasScheduling = document.querySelector('nylas-scheduling');
// Get the scheduler configuration ID from the URL ?config_id=NYLAS_SCHEDULER_CONFIGURATION_ID
const urlParams = new URLSearchParams(window.location.search);
// If not config_id exists, throw a console.error
if (!urlParams.has('config_id')) {
console.error('No scheduler configuration ID found in the URL. Please provide a scheduler configuration ID.');
}
// Set the scheduler configuration ID
nylasScheduling.configurationId = urlParams.get('config_id');
</script>
</body>
</html>
import { BrowserRouter, Route, Routes } from "react-router-dom";
import { NylasSchedulerEditor, NylasScheduling } from "@nylas/react";
import "./App.css";
function App() {
// Get the configuration ID from the URL query string
const urlParams = new URLSearchParams(window.location.search);
const configId = urlParams.get("config_id") || "";
return (
<NylasScheduling
configurationId={configId}
/>
);
}
export default App;
-
In the UI, create a Scheduling Page from the Scheduler Editor.
Creating a Scheduling Page creates a corresponding configuration.- The Scheduling Page's URL includes the configuration ID.
- You can also make a
GET /v3/grants/<NYLAS_GRANT_ID>/scheduling/configurations
request to retrieve the configuration ID.
Create a configuration using the Scheduler API
You can make a POST /v3/grants/<NYLAS_GRANT_ID>/scheduling/configurations
request to create a configuration.
By default, Nylas creates a private configuration that requires a session. To create a public configuration without a session, set requires_session_auth
to false
. See Scheduler configurations for more information.
The following example creates a public configuration. The response includes the ID
of the Configuration object.
curl --request POST \
--url "https://api.us.nylas.com/v3/grants/<NYLAS_GRANT_ID>/scheduling/configurations" \
--header 'Accept: application/json' \
--header 'Authorization: Bearer <NYLAS_API_KEY>' \
--header 'Content-Type: application/json' \
--data '{
"version": "1.0.0",
"requires_session_auth": false,
"participants": [
{
"name": "Test",
"email": "nylas_test_8",
"is_organizer": true,
"availability": {
"calendar_ids": [
"primary"
]
},
"booking": {
"calendar_id": "primary"
}
}
],
"availability": {
"duration_minutes": 30
},
"event_booking": {
"title": "My test event"
}
}'
{
"ID": "AAAA-BBBB-1111-2222",
"version": "1.0.0",
"participants": [
{
"name": "Nylas",
"email": "[email protected]",
"is_organizer": true,
"availability": {
"calendar_ids": [
"primary"
]},
"booking": {
"calendar_id": "primary"
}}],
"availability": {
"duration_minutes": 30
},
"event_booking": {
"title": "My test event"
}
}
(Optional) Create a session
💡 If you created a public configuration in the previous step, you can skip to the next step.
Next, create a session by making a POST /v3/scheduling/sessions
request. When you create a session, you must include the Configuration object ID that you generated in the previous step. Nylas recommends you set a Time-to-Live (TTL) value for each session, and refresh the sessions as they expire.
The response includes the ID of the session.
curl --request POST \
--url "https://api.us.nylas.com/v3/scheduling/sessions" \
--header 'Accept: application/json' \
--header 'Authorization: Bearer <NYLAS_API_KEY>' \
--header 'Content-Type: application/json' \
--data '{
"configuration_id": "AAAA-BBBB-1111-2222",
"time_to_live": 10
}'
{
"request_id": "5fa64c92-e840-4357-86b9-2aa364d35b88",
"data": {
"session_id": "XXXX-YYYY-1111-2222"
}
}
Set up the Scheduler UI Components
💡 If you created a configuration using the Scheduler Editor, you can skip to the next step.
Set up the Scheduler Editor Component
To modify the configuration in the UI, you can set up the Scheduler Editor component.
Set the nylasSessionsConfig
parameter. The Scheduler Editor Component uses the Hosted authentication details in nylasSessionsConfig
to interact with the Nylas APIs.
<html>
<head>
<script type="module" src="https://unpkg.com/@nylas/web-elements@latest"></script>
</head>
<body>
<nylas-scheduler-editor />
<script type="module">
const schedulerEditor = document.querySelector("nylas-scheduler-editor");
schedulerEditor.nylasSessionsConfig = {
clientId: 'NYLAS_CLIENT_ID', // Replace with your Nylas client ID
redirectUri: `${window.location.origin}/scheduler-editor`,
domain: 'https://api.us.nylas.com/v3', // or 'https://api.eu.nylas.com/v3' for EU data center
hosted: true,
accessType: 'offline',
}
</script>
</body>
</html>
import React from 'react';
import { NylasSchedulerEditor } from "@nylas/react";
function App() {
return (
<NylasSchedulerEditor
nylasSessionsConfig={{
clientId: "NYLAS_CLIENT_ID", // Replace with your Nylas client ID
redirectUri: `${window.location.origin}/scheduler-editor`,
domain: "https://api.us.nylas.com/v3", // or 'https://api.eu.nylas.com/v3' for EU data center
hosted: true,
accessType: 'offline'
}}
/>
);
}
export default App;
Set up the Scheduling Component
The Scheduling Component requires either a sessionId
(private configuration) or a configurationId
(public configuration) to load the Scheduling Page with the associated configuration.
By default, the Scheduling Component renders all of its sub-components (mode="app"
). If nothing is configured in the app
mode, Scheduler follows the standard booking flow. See Using the Scheduling Component for more information about configuration modes.
<html>
<head>
<script type="module" src="https://unpkg.com/@nylas/web-elements@latest"></script>
</head>
<body>
<nylas-scheduling
sessionId="<SCHEDULER_SESSION_ID>" /> <!--OR configurationId="<SCHEDULER_CONFIG_ID>"-->
</body>
</html>
import React from 'react';
import { NylasSchedulerEditor } from "@nylas/react";
function App() {
return (
<NylasScheduling
sessionId={"<SCHEDULER_SESSION_ID>"} // OR configurationId={"<SCHEDULER_CONFIG_ID>"}
/>
);
}
export default App;
Set up rescheduling and cancelling options
Booking confirmations and email notifications can include buttons that allow the participant to reschedule or cancel the booking. If they click one of the buttons, they're redirected to the corresponding page where they can make changes to their booking.
To set up the rescheduling and cancelling options, you need to create the rescheduling and cancelling pages and then add URLs to the configuration.
Create rescheduling and cancelling pages
Before you can include the option to reschedule or cancel a booking, you must create corresponding pages and set up their URLs.
To create a page to reschedule an existing booking, add the rescheduleBookingRef
property to the Scheduling Component and set it to the booking reference that you want to update.
To create a page to cancel an existing booking, include the cancelBookingRef
property to the Scheduling Component and set it to the booking reference that you want to cancel.
🔍 You can find the booking reference in the URL of the email notification. For example, https://example.com/mail/#inbox/FMfcgzGxSlVHnZBcBwnfPWvtvjZjpqbZ
.
<NylasScheduling
sessionId={"<SCHEDULER_SESSION_ID>"} // OR configurationId={"<SCHEDULER_CONFIG_ID>"}
rescheduleBookingRef="<BOOKING_REFERENCE>"
/>
<NylasScheduling
sessionId={"<SCHEDULER_SESSION_ID>"} // OR configurationId={"<SCHEDULER_CONFIG_ID>"}
cancelBookingRef="<BOOKING_REFERENCE>"
/>
Add URLs to the configuration
You can add the URLs to the configuration by modifying the Scheduler Editor Component or using the Scheduler API.
Modify the Scheduler Editor Component
To update the configuration in the Scheduler Editor Component, set the rescheduling_url
and cancelling_url
parameters. These should correspond with the URLs of the rescheduling and cancelling pages you created.
<html>
<head>
<script type="module" src="https://unpkg.com/@nylas/web-elements@latest"></script>
</head>
<body>
<nylas-scheduler-editor />
<!-- Configure the Nylas Scheduler Editor Component -->
<script type="module">
const schedulerEditor = document.querySelector('nylas-scheduler-editor');
schedulerEditor.nylasSessionsConfig = {
clientId: 'NYLAS_CLIENT_ID', // Replace with your Nylas client ID
redirectUri: `${window.location.origin}/scheduler-editor`,
domain: 'https://api.us.nylas.com/v3', // or 'https://api.eu.nylas.com/v3' for EU data center
hosted: true,
accessType: 'offline',
}
schedulerEditor.defaultSchedulerConfigState = {
selectedConfiguration: {
scheduler: { // The callback URLs to be set in email notifications
rescheduling_url: `${window.location.origin}/reschedule/<BOOKING_REFERENCE>`, // The URL of the email notification includes the booking reference
cancellation_url: `${window.location.origin}/cancel/<BOOKING_REFERENCE>`
},
},
};
</script>
</body>
</html>
import React from 'react';
import { NylasSchedulerEditor } from "@nylas/react";
function App() {
return (
<NylasSchedulerEditor
nylasSessionsConfig={{
clientId: "<NYLAS_CLIENT_ID>", // Replace with your Nylas client ID
redirectUri: `${window.location.origin}/scheduler-editor`,
domain: "https://api.us.nylas.com/v3", // or 'https://api.eu.nylas.com/v3' for EU data center
hosted: true,
accessType: 'offline',
}}
defaultSchedulerConfigState={{
selectedConfiguration: {
scheduler: { // The callback URLs to be set in email notifications
rescheduling_url:`${window.location.origin}/reschedule/<BOOKING_REFERENCE>`, // The URL of the email notification includes the booking reference
cancellation_url:`${window.location.origin}/cancel/<BOOKING_REFERENCE>`
}
}
}}
/>
);
}
export default App;
Add URLs to configuration using the Scheduler API
To update the configuration, make a PUT /v3/grants/<NYLAS_GRANT_ID>/scheduling/configurations/<SCHEDULER_CONFIG_ID>
request with scheduler.rescheduling_url
and scheduler.cancelling_url
.
curl --request PUT \
--url "https://api.us.nylas.com/v3/grants/<NYLAS_GRANT_ID>/scheduling/configurations/<SCHEDULER_CONFIG_ID>" \
--header 'Accept: application/json' \
--header 'Authorization: Bearer <NYLAS_API_KEY>' \
--header 'Content-Type: application/json' \
--data '{
"version": "1.0.1",
"scheduler": {
"rescheduling_url": "https://www.example.com/reschdule/<BOOKING_REFERENCE>",
"cancellation_url": "https://www.example.com/cancel/<BOOKING_REFERENCE>"
}
}'
{
"ID": "AAAA-BBBB-1111-2222",
"version": "1.0.1",
"participants": [
{
"name": "Nylas",
"email": "[email protected]",
"is_organizer": true,
"availability": {
"calendar_ids": [
"primary"
]},
"booking": {
"calendar_id": "primary"
}}],
"availability": {
"duration_minutes": 30
},
"event_booking": {
"title": "My test event"
},
"scheduler": {
"rescheduling_url": "https://www.example.com/reschduling/<BOOKING_REFERENCE>",
"cancellation_url": "https://www.example.com/cancelling/<BOOKING_REFERENCE>"
}
}
(Optional) Style components using CSS shadow parts
The Scheduler UI Components support CSS shadow parts (::part()
) for a higher level of UI customization. CSS shadow parts allow you to style certain parts of a web component without having to modify its internal structure or styles directly. For more information on available shadow parts, see the Scheduler UI Components reference documentation.
The following CSS sets the background and foreground colors for a component on the Scheduling Page.
/* Your stylesheet */
nylas-scheduling::part(ndp__date--selected) {
background-color: #2563EB;
color: #FFFFFF;
}
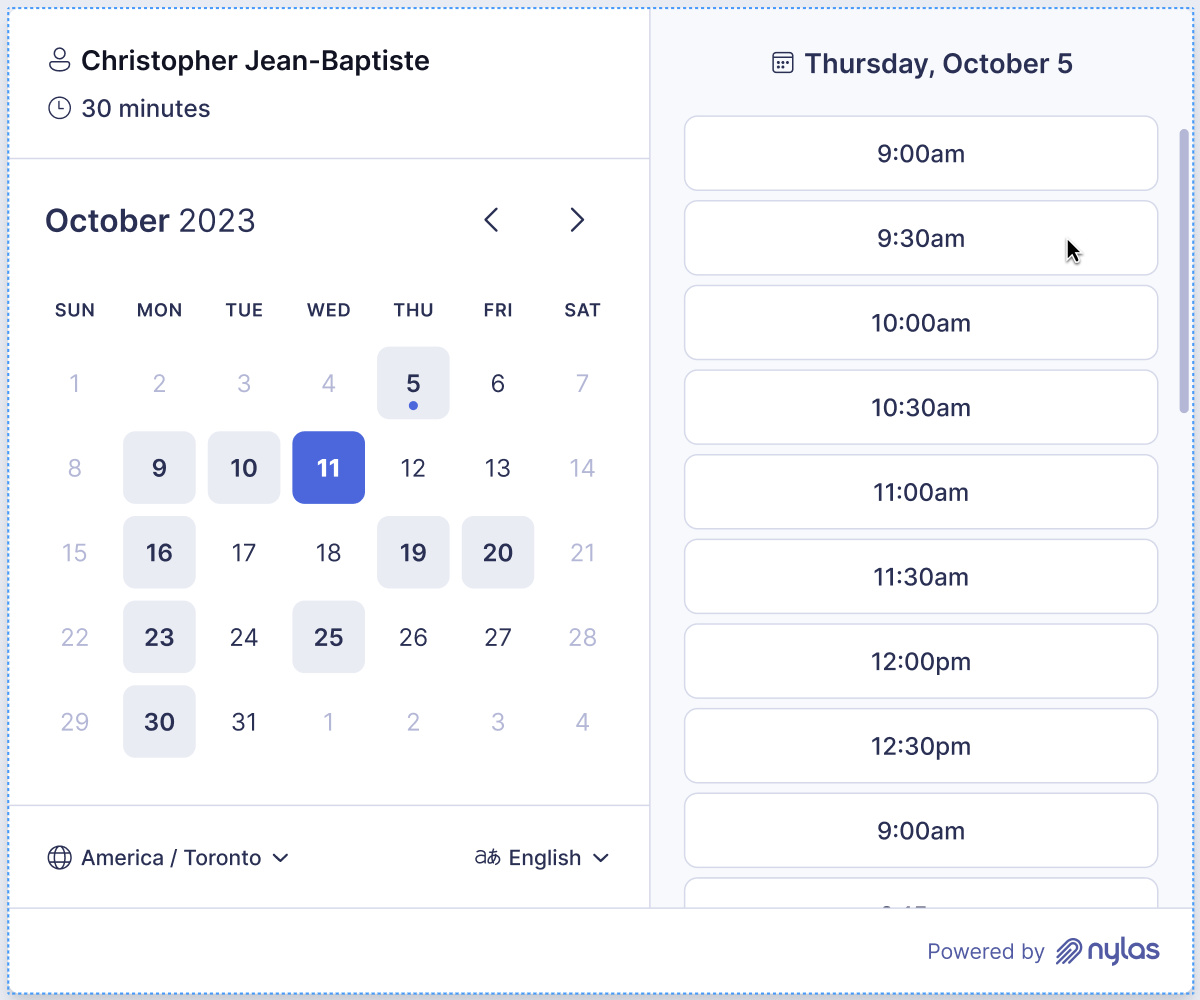
(Optional) Disable email notifications
By default, Nylas sends email notifications to all attendees when a booking is created, rescheduled, or cancelled. To disable email notifications, set disable_emails
to true
in the configuration.
What's next?
- Check out the Scheduler UI Components reference documentation and the Scheduler API reference documentation.
- Learn more about how to use the Scheduler API.
- Learn more about how to use the Scheduling Component.
- Learn more about how to use the Scheduler Editor Component.